Stream Golf
Game Development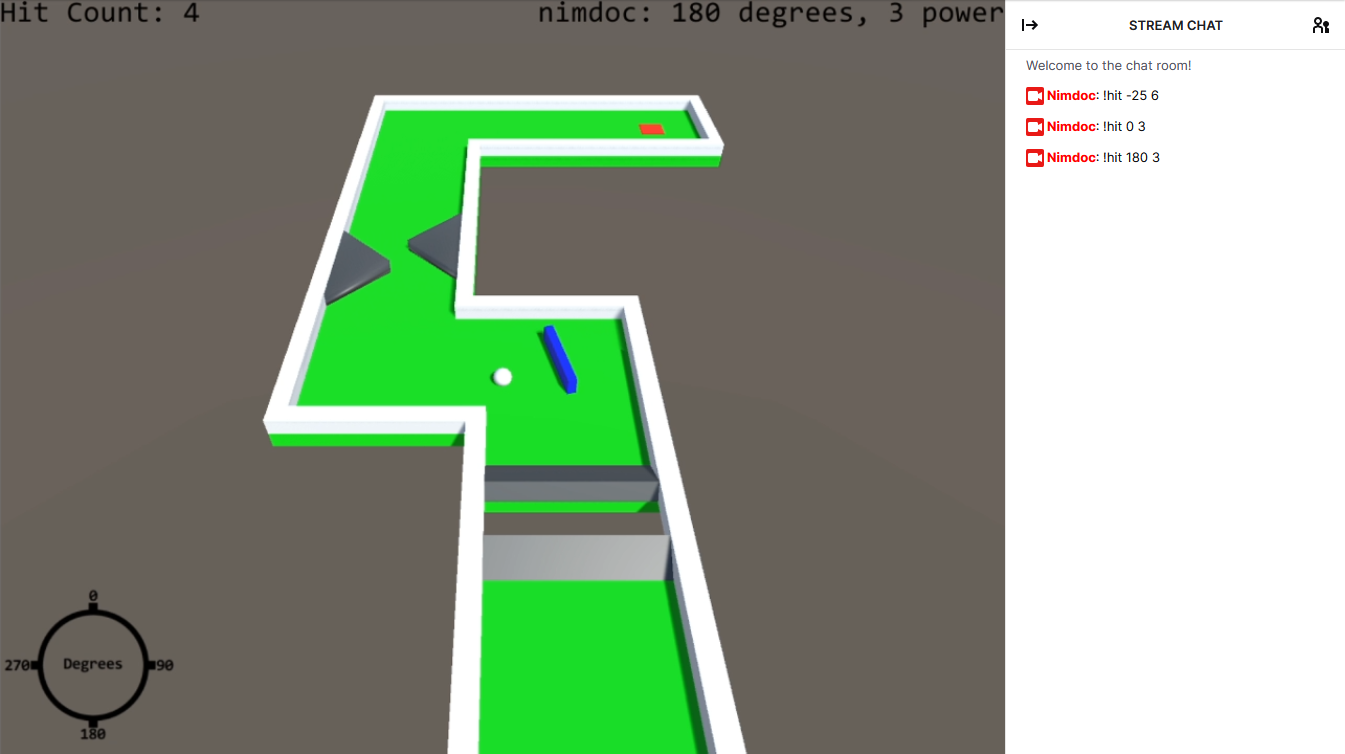
Stream Golf is a multiplayer golf game controlled by users in Twitch chat. You can download it from my Github below below:
You will need to generate an access token for your own Twitch bot and then put your information into the settings of the game.
Development
When I started developing this game I knew that I didn't want the courses to be hard coded. Hard coding levels would take a good amount of effort, and I would need to make a lot of them so that players wouldn't be bored. So instead I decided to procedurally generate the levels. But the levels couldn't be too random. Since the player's camera would only point in one direction due to the controls, I would need the endpoint to definitely be ahead of the start point so that players wouldn't get confused on where to go.
Map Generation
I wanted the course to start at a defined location and for the end of the course to definitely be straight ahead a certain distance. But I didn't want the path of the course to always go straight to the end, I wanted there to be some variety. I decided to use Markov Decision Process policies. Normally in MDPs you have states that have positive values, negative values, or no value at all. Below is a simple map I drew where the grid spaces are the states:
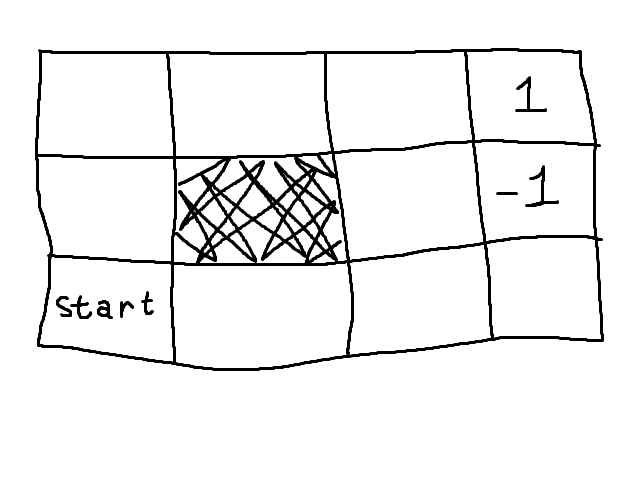
A policy is a set of actions that the algorithm will take when in certain states (or squares as shown above). For example the set of actions could look like this:
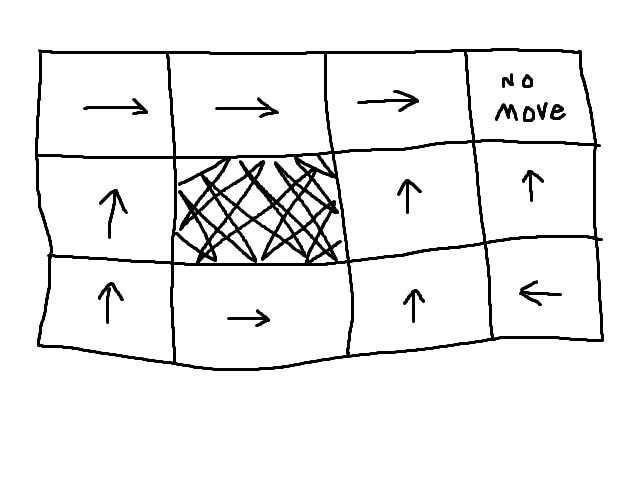
In the case of the game though, I don't have multiple spaces that have positive or negative values. I only have one space that matters and that is the end space, or the hole in golf. So I don't need to do any fancy MDP algorithms like Value Iteration or Policy Iteration. Instead I can just determine what action I need to take by seeing what move (up, down, left, or right) will make the distance to the end shorter. So I wrote an algorithm that does just that for an 11 by 11 grid, and here's the output of the policy from Powershell:
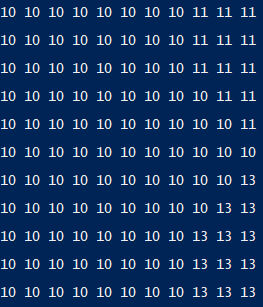
The number are just constants for:
- Up - 13
- Down - 11
- Left - 12
-
Right - 10
The map is simple, and could possibly be hard coded, but being generated allows be to change the size of the map if I wanted to. So now that I have a policy generated, I can actually start to generate the golf course map. So the way I do that is I program an agent to make it's way to the end. The agent will look at the policy and determine which direction to head towards to get to the end. But every time the agent tries to move, there will be a chance the the agent moves in the wrong direction. That is where the randomness of the golf course comes in. Here are a couple golf course maps that were generated in Powershell:
The values in these maps are:
- 0 - Nothing
- 1 - A space in golf
- 2 - The Start
-
3 - The end
After implementing this algorithm in Unity it looked like this:
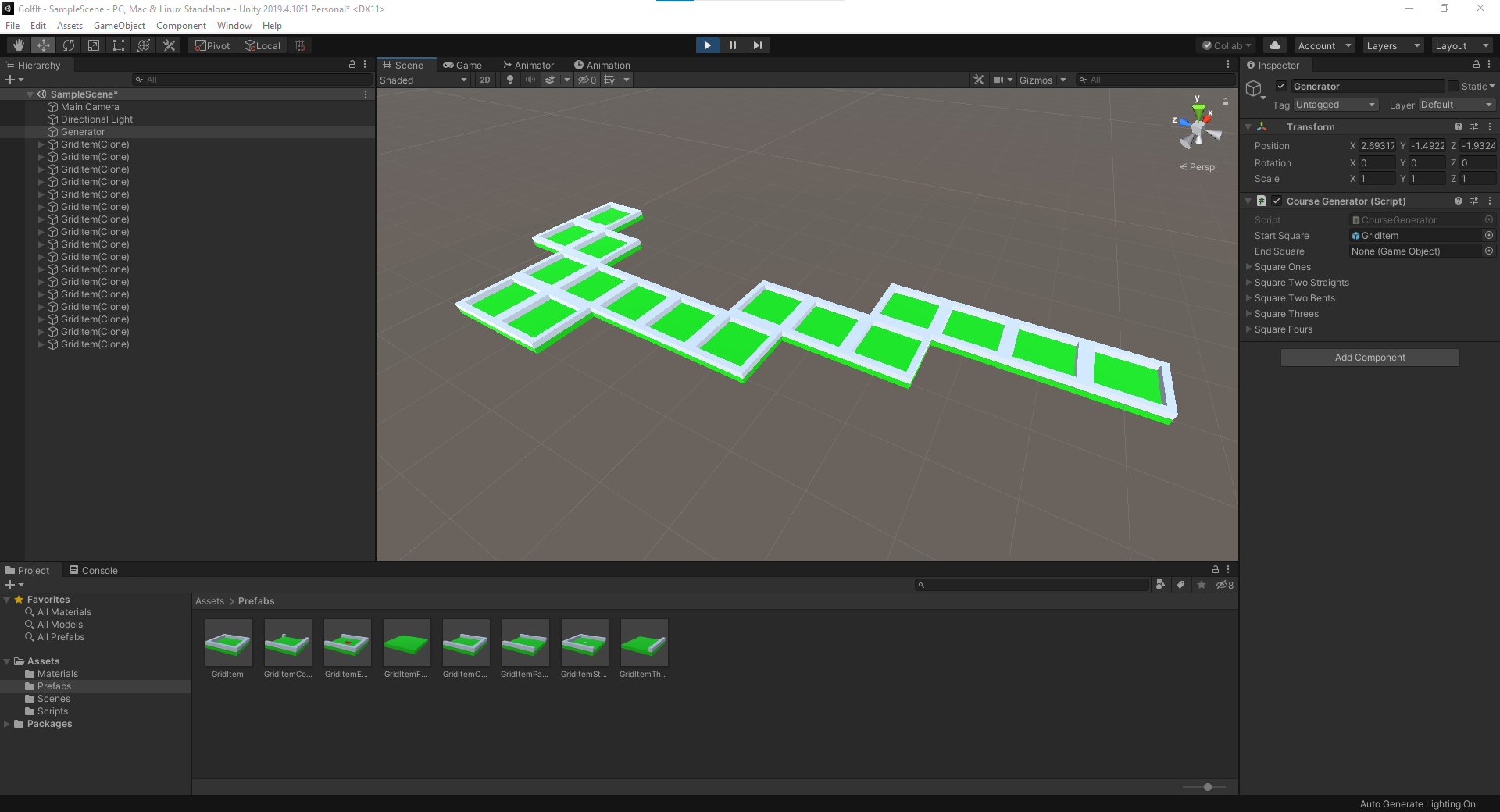
Then after adding in the corners, straight aways, and other grid pieces, rotating them correctly, and then adding some variety, I ended up with maps like this:
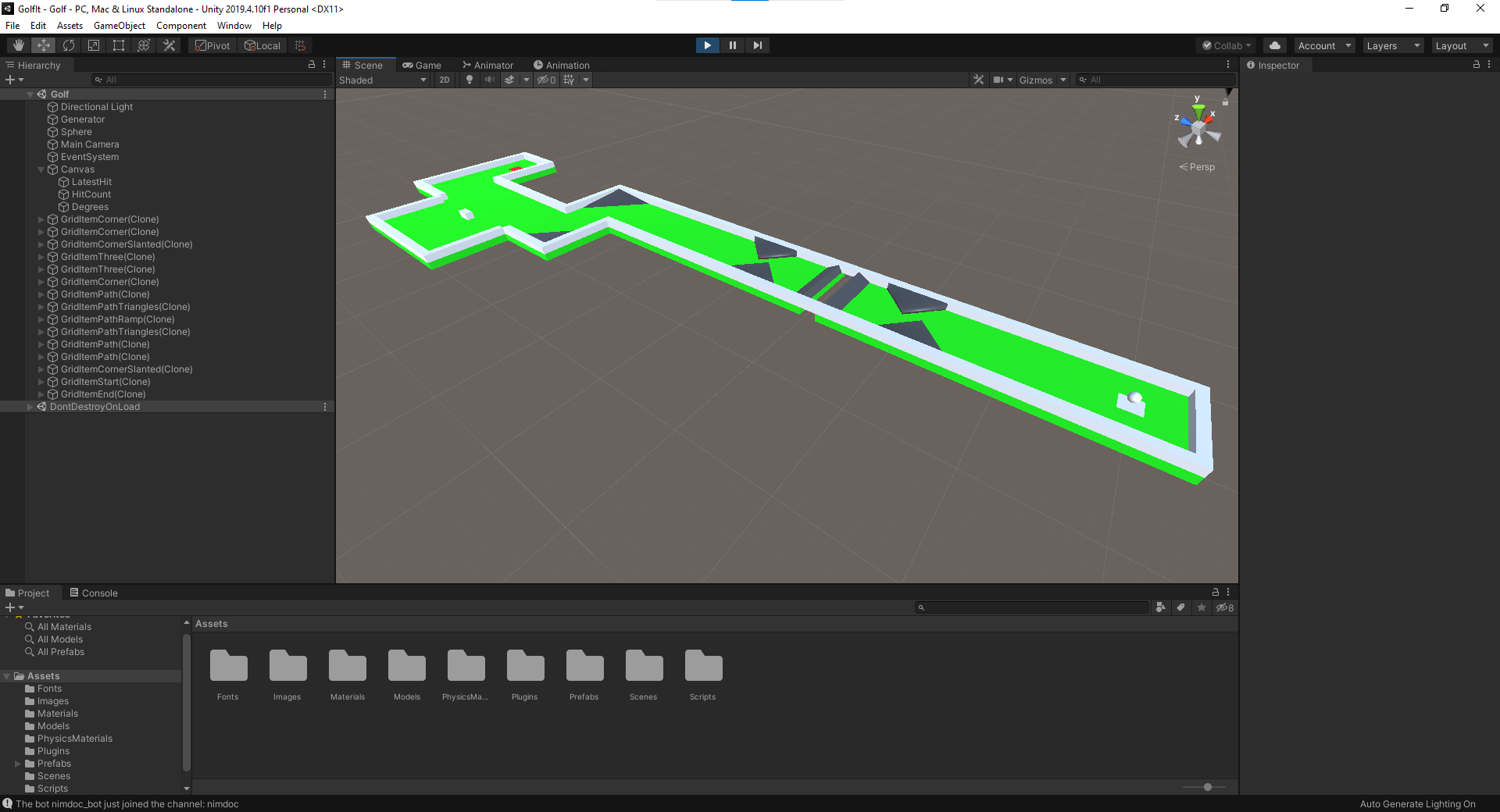
Player Input
Implementing player input through Twitch chat was very simple using TwitchLib.Unity. I setup up a ball that was controlled by typing in Twitch chat a command like:
!hit 90 5
This would hit the ball to the right with 5 "power". Power being arbitrary.
Polish
I just added a minimal amount of polish such as:
- A main menu
- A settings menu
- A compass in the game
- A message for who last hit the ball
-
How many hits the players have input for the current game
I'd add more polish if the game got popular but for now I want to work on other stuff.
Conclusion
I had a lot of fun developing this small game. I designed it because I was thinking about streaming a game for fun on Twitch, but I don't have much to talk about. So if someone stopped in to watch the stream, they could at least play golf in the corner. Along designing this game I learned more about Unity and I learned more about the syntax of C#.