Hosting Websites & Services Without a Static IP Address
Web Development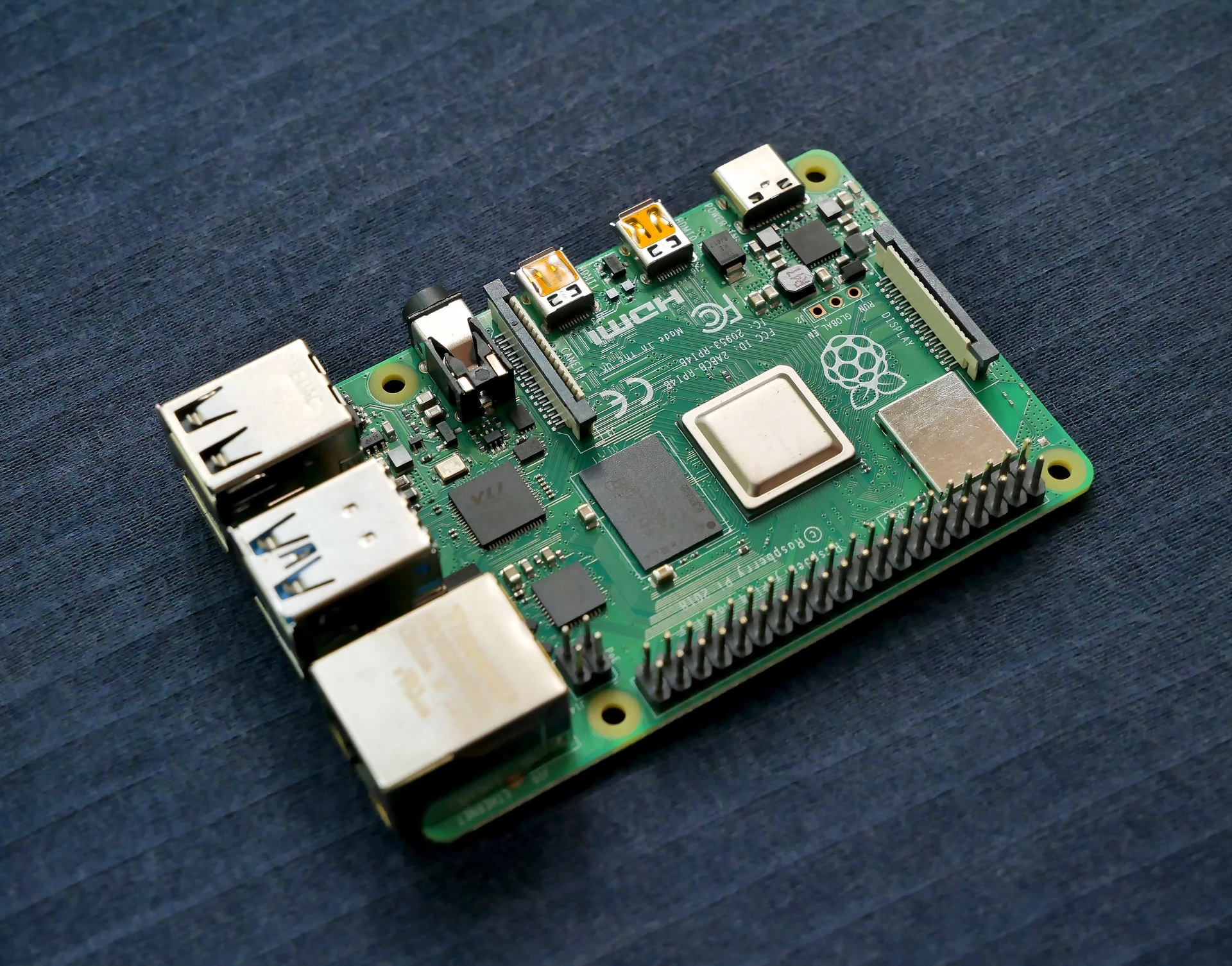
Back in 2012 my mother bought me the very first Raspberry Pi. It’s an awesome tiny computer, and I still have it today. Over the years I did a bunch of stuff on it, mainly web programming. I setup an e-mail server and learned how stringent the anti spam measures are; I setup a forum on it so classmates and I could coordinate meetings and project files for a class; And I hosted several hobby websites of mine.
One big part of all of these projects was being able to connect to the Raspberry Pi from outside my home network. Port forwarding the home router was simple enough. But I also wanted domain names for all of these projects, cause of course I don’t want to tell someone hey go to your internet browser and type “98.49.207.48” or something. Plus residential internet plans usually assign IP addresses via DHCP.
For a while I just had my domains point to whatever my IP address at home was at the time. I used to live in a more rural part of America, so the IP addresses didn’t change that often for residential connections. So I’d only have to go and update my DNS settings for my domains once every couple months, at most. But then I moved to an apartment in a bigger city for college, and I brought my Raspberry Pi with me. A lot more people in the city obviously, and with that a lot more IP address changes. My IP address in my new apartment would change at least once a week, so updating my DNS settings manually was going to be an annoying hassle for me.
There are a couple DNS handling services out there like Dyn DNS. But those cost money or have limitations. If I was gonna start to spend money on my hobby websites I might as well buy server space somewhere cheap instead. But I was a stingy poor college student so that wasn’t going to happen.
So I looked into my domain registrar, name.com, and saw that it had an API. It was pretty simple and so I made a small bash script that would check what my home IP address was, and then send an API request to name.com to update my DNS settings in the event that my home IP address changed.
#!/bin/bash ip="$(host myip.opendns.com resolver1.opendns.com | grep "myip.opendns.com has" | awk '{print $4}')" echo "Current IP:" echo $ip stored_ip=`cat stored_ip.txt` echo "Stored IP: " echo $stored_ip if [ $ip != $stored_ip ] then curl -u '[USERNAME]:[API_KEY]' 'https://api.name.com/v4/domains/[MY_DOMAIN]/records/[RECORD_ID]' -X PUT --data '{"host":"","type":"A","answer":"'"$ip"'","ttl":300}' sleep 5 curl -u '[USERNAME]:[API_KEY]' 'https://api.name.com/v4/domains/[MY_DOMAIN]/records/[RECORD_ID]' -X PUT --data '{"host":"www","type":"A","answer":"'"$ip"'","ttl":300}' echo $ip > stored_ip.txt insert=$ip insert+="," insert+="$(date +%Y-%m-%dT%H:%M:%S)" echo $insert >> ip_log.csv echo "Updated domain IP address." fi
I had this script run on a cron every 5 minutes, and it worked great for my needs.
This isn’t the first version of the script actually. In my first version instead of storing the current IP in a file, I naively had it ping name.com to check what the IP address was in their DNS records. And apparently pinging name.com every 5 minutes was over their rate limit, so they cut me off for the rest of the month. When I e-mailed them to ask why my requests were failing they told me I went over their rate limit. So I asked what their rate limit was (because it wasn’t in their documentation at the time), and the support person didn’t know. But whatever my rate was (~288 requests a day given my calculations) it was over their limit.
As you can also see in my script above, I logged the time & date when my IP address would change. I still have the log from when I started in April 2018, and mapped it on a calendar. My IP address changed a total of 20 times in 4 months. Then after July 25th it didn’t change until May of the next year. I’m unsure why.
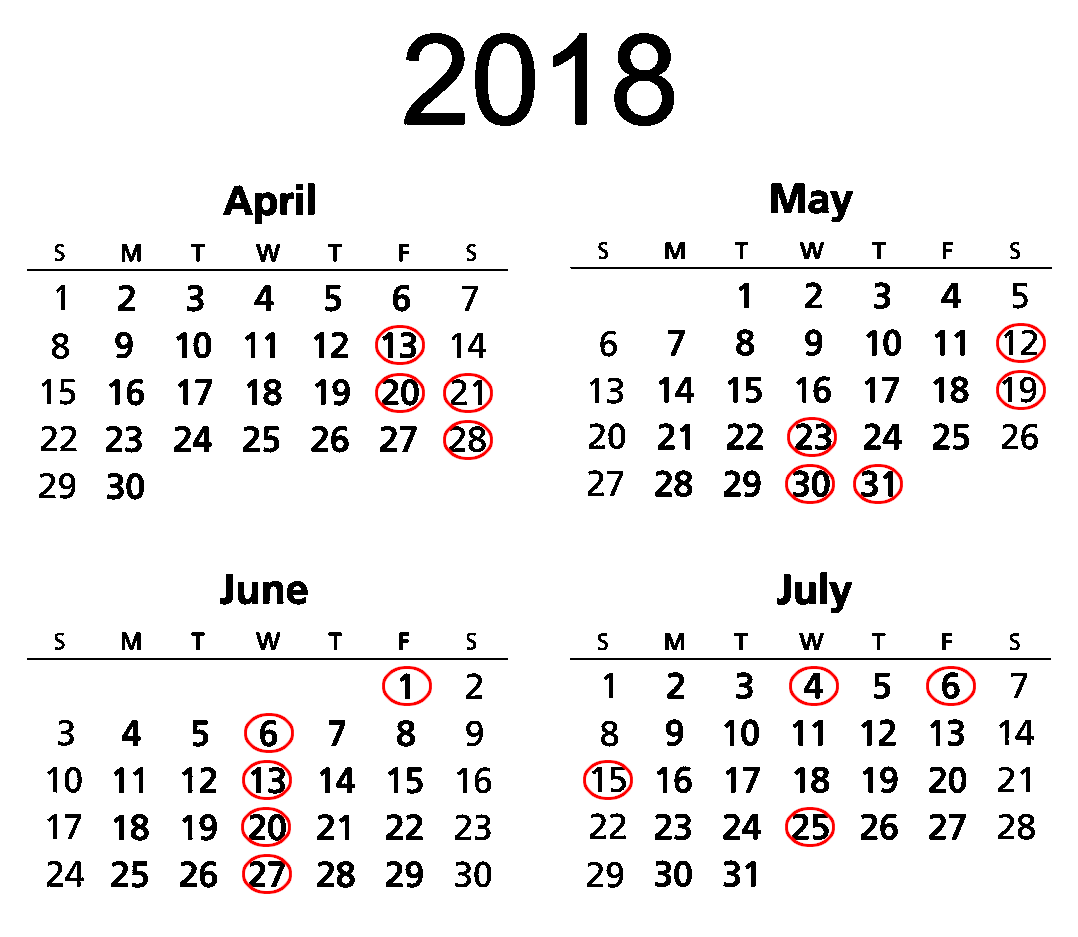
Another nice thing about having a Raspberry Pi automatically updating a domain to point to my home IP address is playing videogames with my friends. A lot of videogames, especially older ones, don’t have automatic match making. Instead you have to host the server yourself and figure out how to have your friends connect themselves. When my friends would go to join my server, there’d be a place to type an IP address. But they could type something like tomscooldomain.com to connect, instead of me having to figure out my IP address and sending it to them.